By: T3
Since: Jul 2017
Licence: MIT
1. Setting up
1.1. Prerequisites
-
JDK
1.8.0_60
or laterHaving any Java 8 version is not enough.
This app will not work with earlier versions of Java 8. -
Eclipse IDE
-
e(fx)clipse plugin for Eclipse (Do the steps 2 onwards given in this page)
-
Buildship Gradle Integration plugin from the Eclipse Marketplace
-
Checkstyle Plug-in plugin from the Eclipse Marketplace
1.2. Importing the project into Eclipse
-
Fork this repo, and clone the fork to your computer
-
Open Eclipse (Note: Ensure you have installed the e(fx)clipse and buildship plugins as given in the prerequisites above)
-
Click
File
>Import
-
Click
Gradle
>Gradle Project
>Next
>Next
-
Click
Browse
, then locate the project’s directory -
Click
Finish
|
1.3. Configuring checkstyle
-
Click
Project
→Properties
→Checkstyle
→Local Check Configurations
→New…
-
Choose
External Configuration File
underType
-
Enter an arbitrary configuration name e.g. 2Do
-
Import checkstyle configuration file found at
config/checkstyle/checkstyle.xml
-
Click OK once, go to the
Main
tab, use the newly imported check configuration. -
Tick and select
files from packages
, clickChange…
, and select theresources
package -
Click OK twice. Rebuild project if prompted
Click on the files from packages text after ticking in order to enable the Change… button
|
1.4. Troubleshooting project setup
Problem: Eclipse reports compile errors after new commits are pulled from Git
-
Reason: Eclipse fails to recognize new files that appeared due to the Git pull.
-
Solution: Refresh the project in Eclipse: Right click on the project (in Eclipse package explorer), choose
Gradle
→Refresh Gradle Project
.
Problem: Eclipse reports some required libraries missing
-
Reason: Required libraries may not have been downloaded during the project import.
-
Solution: Run tests using Gradle once (to refresh the libraries).
1.5. Updating documentation
After forking the repo, links in the documentation will link to the wrong repo. You should replace the URL in the variable repoURL
in DeveloperGuide.adoc with the URL of your fork.
1.6. Coding style
We follow oss-generic coding standards.
2. Design
2.1. Architecture
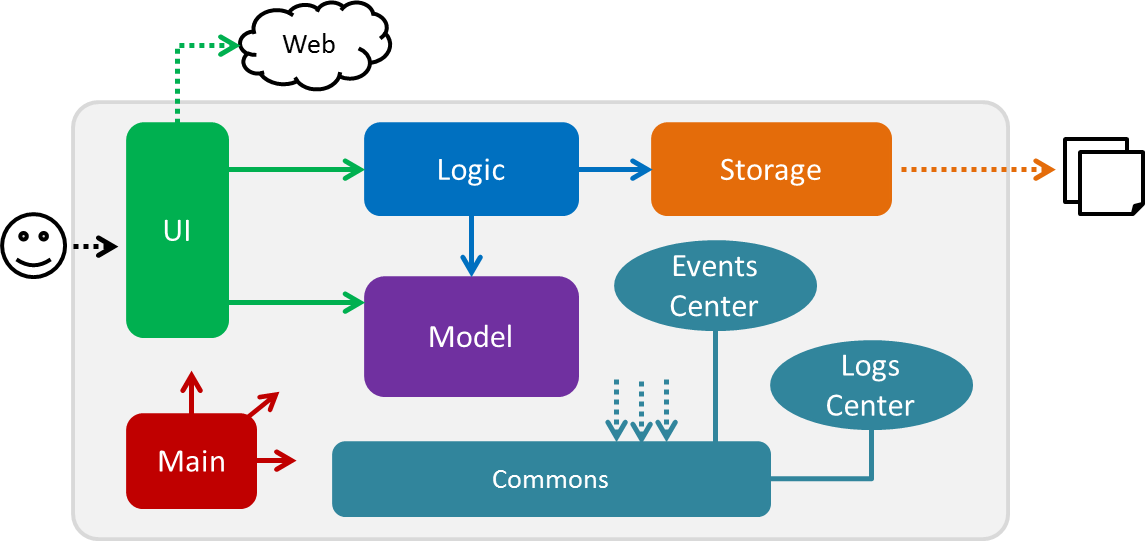
Figure 2.1.1 : Architecture Diagram
The Architecture Diagram given above explains the high-level design of 2Do. Given below is a quick overview of each component.
The .pptx files used to create diagrams in this document can be found in the diagrams folder. To update a diagram, modify the diagram in the pptx file, select the objects of the diagram, and choose Save as picture .
|
Main
has only one class called MainApp
. It is responsible for,
-
At app launch: Initializes the components in the correct sequence, and connects them up with each other.
-
At shut down: Shuts down the components and invokes cleanup method where necessary.
Commons
represents a collection of classes used by multiple other components. Two of those classes play important roles at the architecture level.
-
EventsCenter
: This class (written using Google’s Event Bus library) is used by components to communicate with other components using events (i.e. a form of Event Driven design) -
LogsCenter
: Used by many classes to write log messages to 2Do's log file.
The rest of the App consists of four components.
Each of the four components
-
Defines its API in an
interface
with the same name as the Component. -
Exposes its functionality using a
{Component Name}Manager
class.
For example, the Logic
component (see the simplified class diagram given below) defines it’s API in the Logic.java
interface and exposes its functionality using the LogicManager.java
class.
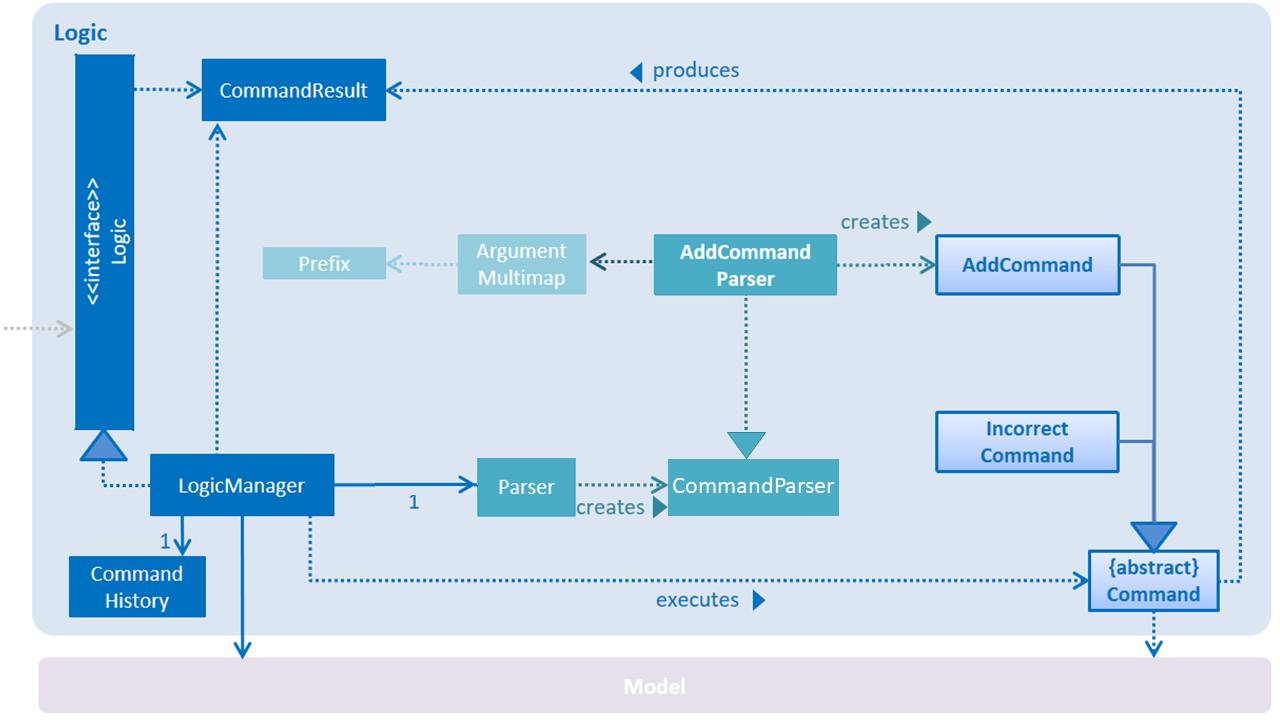
Figure 2.1.2 : Simplified class Diagram of the Logic Component
Events-Driven nature of the design
The Sequence Diagram below shows how the components interact for the scenario where the user issues the command delete 1
.
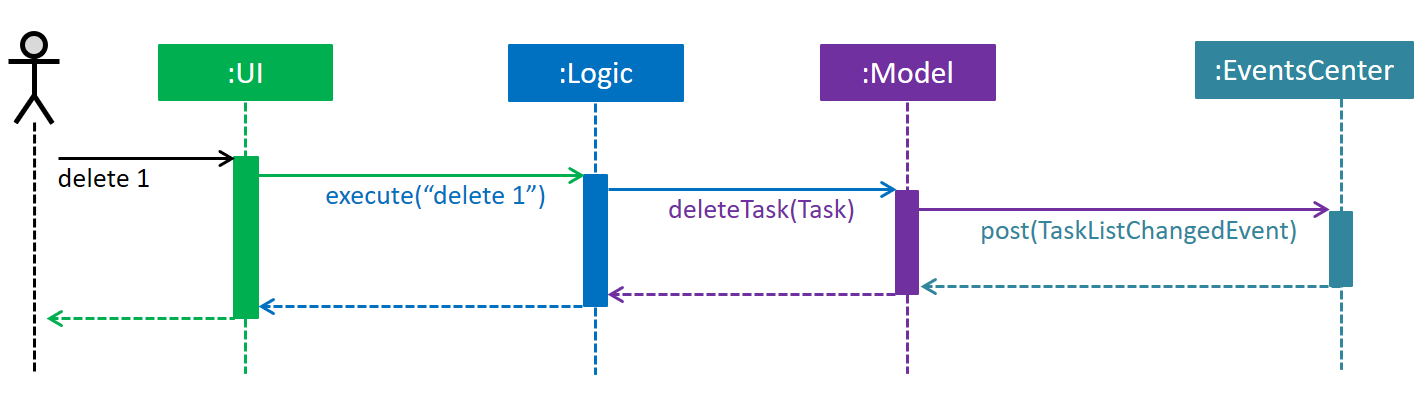
Figure 2.1.3a : Component interactions for delete 1
command (part 1)
Note how the Model simply raises a TaskBookChangedEvent when the TaskList data are changed, instead of asking the Storage to save the updates to the hard disk.
|
The diagram below shows how the EventsCenter
reacts to that event, which eventually results in the updates being saved to the hard disk and the status bar of the UI being updated to reflect the 'Last Updated' time.
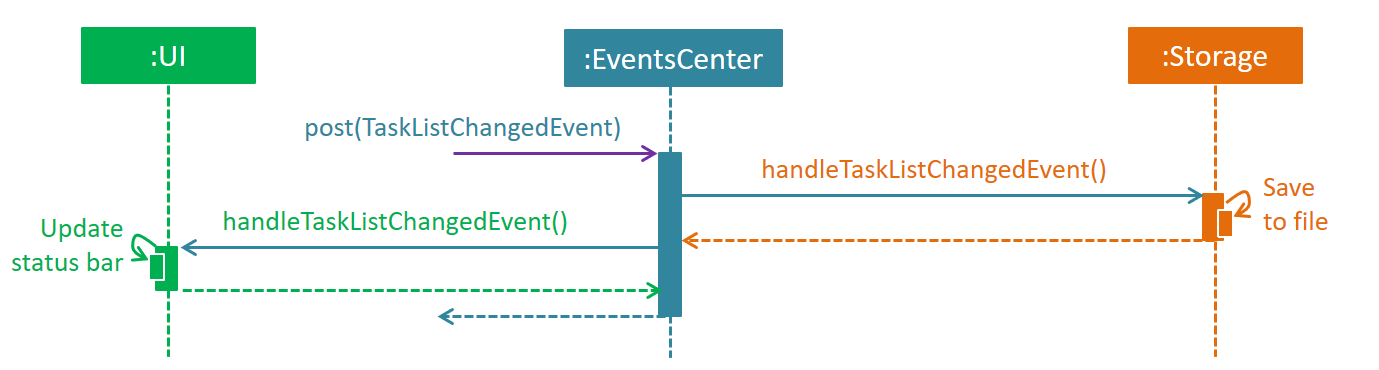
Figure 2.1.3b : Component interactions for delete 1
command (part 2)
Note how the event is propagated through the EventsCenter to the Storage and UI without Model having to be coupled to either of them. This is an example of how this Event Driven approach helps us reduce direct coupling between components.
|
The sections below give more details of each component.
2.2. UI component
Author: Yogamurti Sutanto
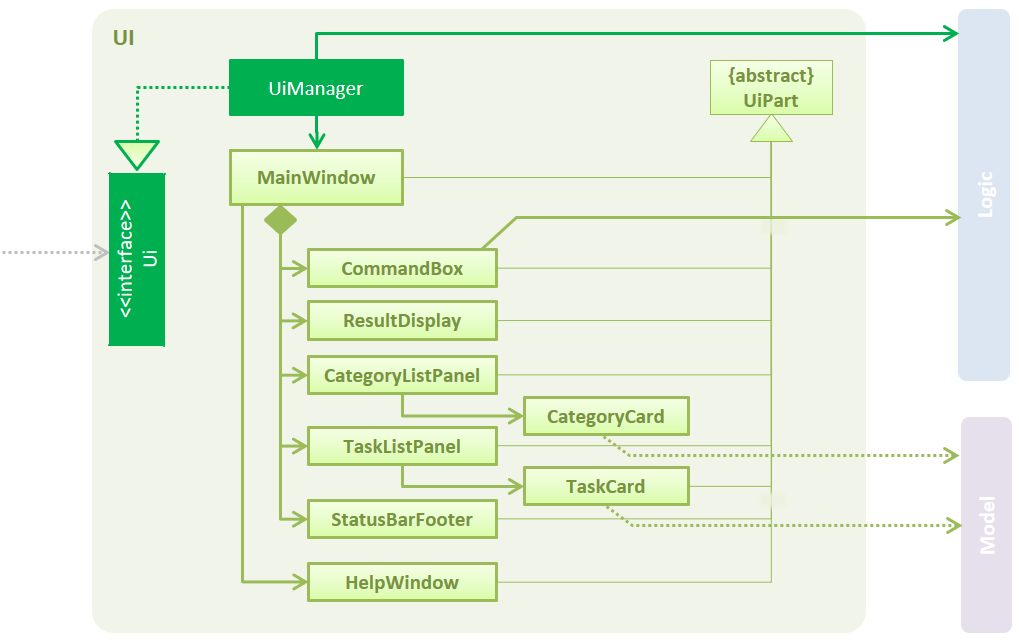
Figure 2.2.1 : Structure of the UI Component
API : Ui.java
The UI
consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, CategoryListPanel
, etc. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses the JavaFx UI framework. The layout of these UI
parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
Model-View-Controller Pattern
2Do decouples its data, presentation, and control logic by separating them into three different components: Model, View and Controller.
-
Model: The
UI
component’sResultDisplay
,CategoryListPanel
,TaskListPanel
,StatusBarFooter
andHelpWindow
binds themselves to some data in theModel
component, which stores and maintains data in 2Do. -
View: The
UI
component is responsible for displaying data, interacting with the user through theLogic
component, and getting automatic data updates from theModel
component. -
Controller: The
UI
component’sCommandBox
is responsible for detecting user command inputs and forwarding them to theLogic
component, which executes the commands.
Observer Pattern
2Do utilizes the observer pattern to avoid direct coupling between the UI
component and other components.
The UI
component’s constituents that depends on data from the Model
component, such as its TaskListPanel
constituent, requires notification whenever the Model
component updates.
This is done by the TaskListChangedEvent.
Whenever the data in the Model
component updates, 2Do notifies its relevant UI
constituents by calling a indicateTaskListChanged() operation, which raises a new TaskListChangedEvent. This event will call the relevant UI
constituents to update their data from the Model
component and display the updated data.
2.3. Logic component
Author: Ang Lang Yi
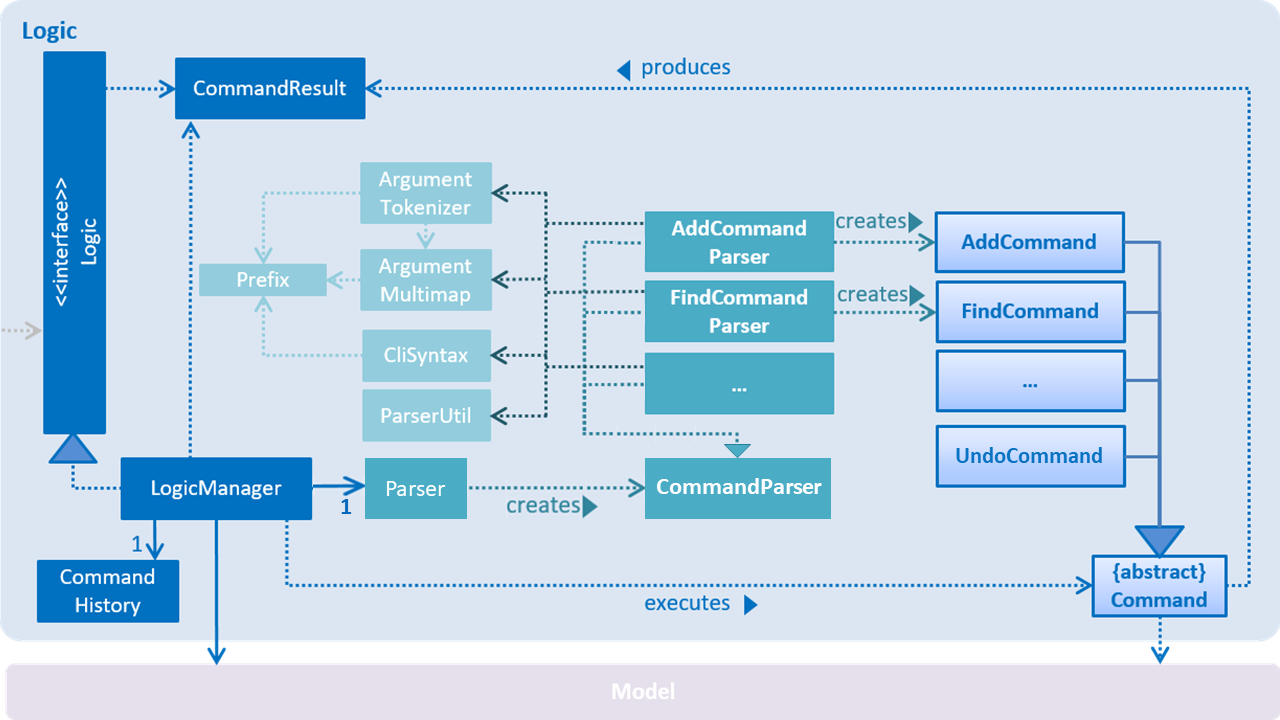
Figure 2.3.1 : Structure of the Logic Component
API :
Logic.java
-
Logic
uses theParser
class to parse the user command. -
The command parsing of each specific command is encapsulated as a
CommandParser
object for the their respective parser objects (e.g. DeleteCommandParser). -
This results in a
Command
object which is executed by theLogicManager
. <<<<<<< HEAD <<<<<<< HEAD -
The command execution can affect the
Model
(e.g. deleting a task) and/or raise events. -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("delete 1")
API call.
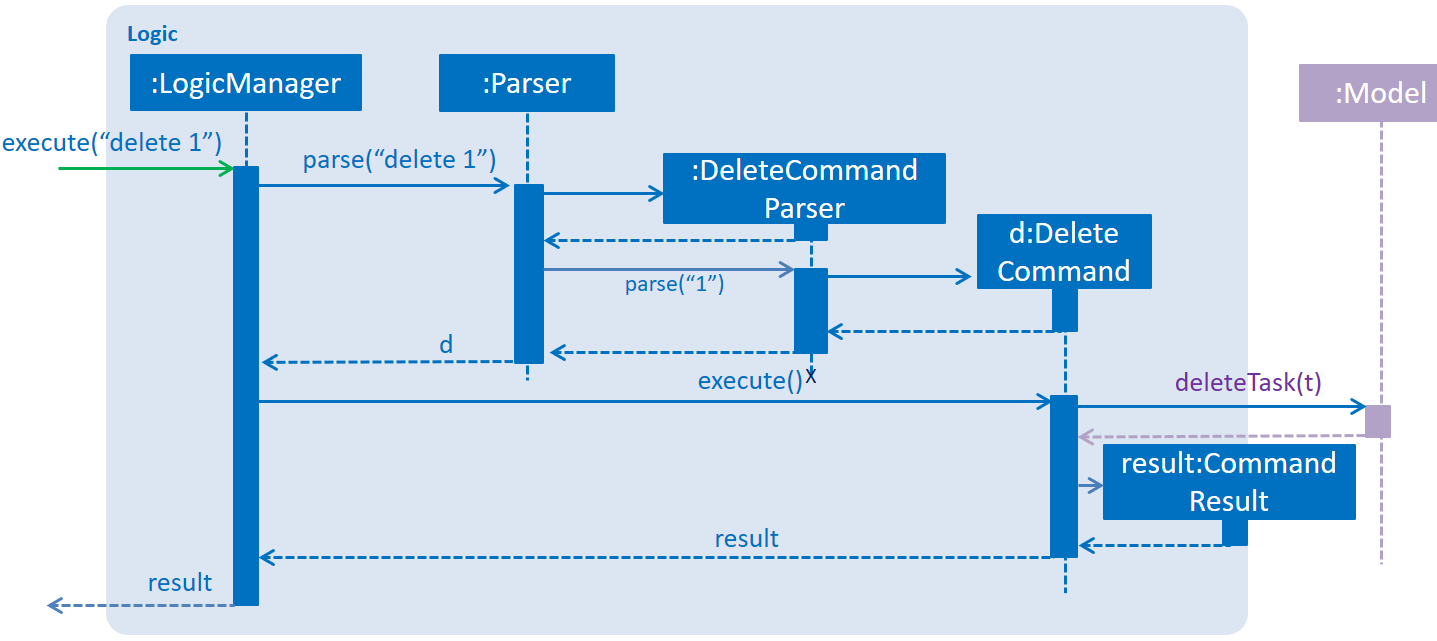
Figure 2.3.1 : Interactions Inside the Logic Component for the delete 1
Command
2.4. Model component
Author: V Narendar Nag
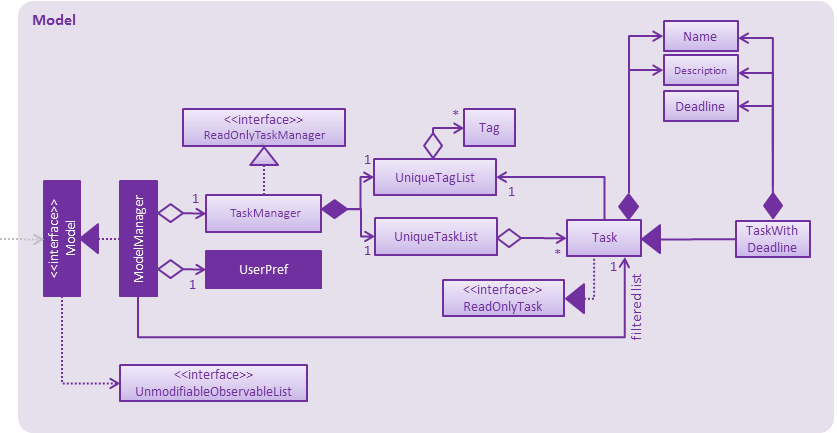
Figure 2.4.1 : Structure of the Model Component
API : Model.java
The Model
,
-
stores a
UserPref
object that represents the user’s preferences. -
stores the Taskbook data.
-
exposes a
UnmodifiableObservableList<ReadOnlyTask>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. -
does not depend on any of the other three components.
2.5. Storage component
Author: Teo Shu Qi
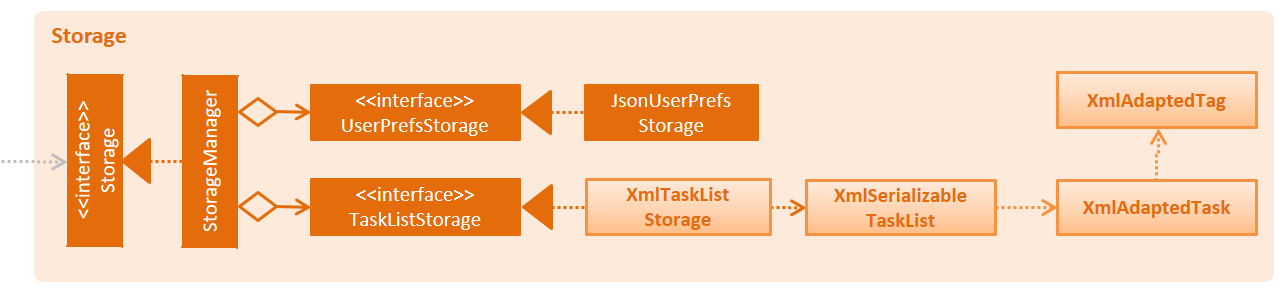
Figure 2.5.1 : Structure of the Storage Component
API : Storage.java
The Storage
component,
-
can save
UserPref
objects in json format and read it back. -
can save the TaskList data in xml format and read it back.
2Do allows users to save their data at a new filepath(File Directory must be available). The filepath can be specified via the Save command and will contain a new 2Do.xml document. StorageMananger will set the new filepath and save it into Config. 2Do will load from and save to the new filepath for current and future sessions until a new filepath is specified. 2Do also allows users to load a TaskList file from previous sessions vias the Load command.
2.6. Common classes
Classes used by multiple components are in the teamthree.twodo.commons
package.
3. Implementation
3.1. Logging
We are using java.util.logging
package for logging. The LogsCenter
class is used to manage the logging levels and logging destinations.
-
The logging level can be controlled using the
logLevel
setting in the configuration file (See Configuration) -
The
Logger
for a class can be obtained usingLogsCenter.getLogger(Class)
which will log messages according to the specified logging level -
Currently log messages are output through:
Console
and to a.log
file.
Logging Levels
-
SEVERE
: Critical problem detected which may possibly cause the termination of the application -
WARNING
: Can continue, but with caution -
INFO
: Information showing the noteworthy actions by the App -
FINE
: Details that is not usually noteworthy but may be useful in debugging e.g. print the actual list instead of just its size
3.2. Configuration
Certain properties of the application can be controlled (e.g App name, logging level) through the configuration file (default: config.json
).
4. Testing
Tests can be found in the ./src/test/java
folder.
In Eclipse:
-
To run all tests, right-click on the
src/test/java
folder and chooseRun as
>JUnit Test
-
To run a subset of tests, you can right-click on a test package, test class, or a test and choose to run as a JUnit test.
Using Gradle:
-
See UsingGradle.adoc for how to run tests using Gradle.
We have two types of tests:
-
GUI Tests - These are System Tests that test the entire App by simulating user actions on the GUI. These are in the
guitests
package. -
Non-GUI Tests - These are tests not involving the GUI. They include,
-
Unit tests targeting the lowest level methods/classes.
e.g.teamthree.twodo.commons.StringUtilTest
-
Integration tests that are checking the integration of multiple code units (those code units are assumed to be working).
e.g.teamthree.twodo.storage.StorageManagerTest
-
Hybrids of unit and integration tests. These test are checking multiple code units as well as how the are connected together.
e.g.teamthree.twodo.logic.LogicManagerTest
-
4.1. Headless GUI Testing
Thanks to the TestFX library we use, our GUI tests can be run in the headless mode. In the headless mode, GUI tests do not show up on the screen. That means the developer can do other things on the Computer while the tests are running. See UsingGradle.adoc to learn how to run tests in headless mode.
4.2. Troubleshooting tests
Problem: Tests fail because NullPointException when AssertionError is expected
-
Reason: Assertions are not enabled for JUnit tests. This can happen if you are not using a recent Eclipse version (i.e. Neon or later)
-
Solution: Enable assertions in JUnit tests as described here. Delete run configurations created when you ran tests earlier.
5. Dev Ops
5.1. Build Automation
See UsingGradle.adoc to learn how to use Gradle for build automation.
5.2. Continuous Integration
We use Travis CI and AppVeyor to perform Continuous Integration on our projects. See UsingTravis.adoc and UsingAppVeyor.adoc for more details.
5.3. Publishing Documentation
See UsingGithubPages.adoc to learn how to use GitHub Pages to publish documentation to the project site.
5.4. Making a Release
Here are the steps to create a new release.
-
Generate a JAR file using Gradle.
-
Tag the repo with the version number. e.g.
v0.1
-
Create a new release using GitHub and upload the JAR file you created.
5.5. Converting Documentation to PDF format
We use Google Chrome for converting documentation to PDF format, as Chrome’s PDF engine preserves hyperlinks used in webpages.
Here are the steps to convert the project documentation files to PDF format.
-
Make sure you have set up GitHub Pages as described in UsingGithubPages.adoc.
-
Using Chrome, go to the GitHub Pages version of the documentation file. e.g. For UserGuide.adoc, the URL will be
https://<your-username-or-organization-name>.github.io/main/docs/UserGuide.html
. -
Click on the
Print
option in Chrome’s menu. -
Set the destination to
Save as PDF
, then clickSave
to save a copy of the file in PDF format. For best results, use the settings indicated in the screenshot below.
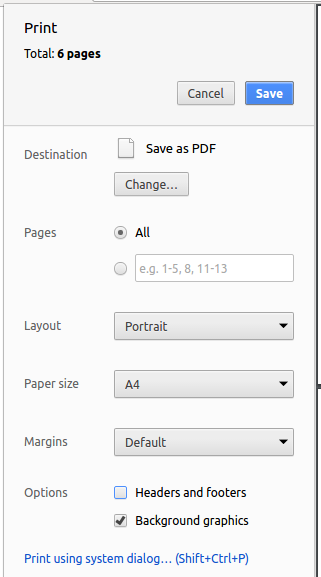
Figure 5.4.1 : Saving documentation as PDF files in Chrome
5.6. Managing Dependencies
A project often depends on third-party libraries. For example, 2Do depends on the Jackson library for XML parsing and the PrettyTime library for natural language handling. Managing these dependencies can be automated using Gradle. Gradle can download the dependencies automatically, which is better than these alternatives:
a. Including those libraries in the repository (this bloats the repository size)
b. Require developers to download those libraries manually (this creates extra work for developers)
Appendix A: User Stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
|
new user |
see usage instructions |
refer to instructions when I forget how to use 2Do |
|
user |
add floating tasks |
manage my floating tasks |
|
user |
manage my tasks with deadlines |
|
|
user |
add events |
manage my events |
|
user |
describe my tasks in detail |
add more information about the tasks, such as the venue |
|
user |
delete tasks |
remove tasks that have been cancelled |
|
user |
edit tasks |
update my task details for unforeseen circumstances, such as postponing a deadline |
|
user |
add deadlines to my floating tasks |
update my floating tasks if they then require a deadline |
|
user |
mark my tasks as completed |
stop being reminded for tasks I have done |
|
user |
mark completed tasks as uncompleted again |
appropriately update my to-do list if the task then requires more follow-up action |
|
user |
list all my uncompleted tasks |
know what I need to do |
|
user |
list all my completed tasks |
review and reflect on the tasks I have done |
|
user |
search for tasks using keywords |
find details of specific tasks |
|
user |
find tasks that are related by some category |
know related tasks easily if I have tagged them with their respective categories |
|
user |
find tasks by deadline |
know which tasks are most urgent |
|
user |
save my to-do list as a readable file |
have a back-up copy of my to-do list and be able to view it across multiple devices |
|
user |
undo the last action |
correct a mistake |
|
user |
have flexible command formats |
execute actions in a more natural manner |
|
user |
add recurring tasks |
not need to add the same task every time it repeats |
|
user |
mark tasks as cannot do |
properly remove tasks that I am unable to complete out of my to-do list |
|
user |
list tasks this week or month |
see only tasks with urgent deadlines to prevent clutter |
|
user |
set a reminder on tasks |
be reminded of my tasks closer to their deadline |
|
user |
have a global reminder setting |
not need to set reminders for each task manually |
|
user |
be alerted of tasks whose deadline has eclipsed but I have not marked as complete |
decide on an appropriate follow-up action |
|
user |
load a to-do list from a storage location |
manage my to-do list from different devices and be able to manage multiple to-do lists |
|
user |
redo a command I have undone |
redo any unintended undo actions |
|
user |
have command shortcuts |
execute actions with ease and speed |
|
user with many tagged tasks |
clear all tasks that have a certain tag |
conveniently clear all related tasks once a project or milestone is completed or cancelled |
|
user |
visualize my tasks as a calendar |
more easily plan my schedule |
|
user |
launch the program quickly, by pressing a keyboard shortcut |
conveniently launch the program |
|
experienced user |
create my own shortcuts for my most frequently used commands |
more conveniently use the program |
|
user with many tasks |
sort tasks in different ways |
gain a better understanding of the tasks at hand |
|
user |
get directions |
get directions to the task’s location, from my current location |
|
user |
block timeslots for events |
stop scheduling events with conflicting timings |
|
user |
unblock timeslots that have been blocked |
schedule other events in that timeslot |
|
user |
synchronize my tasks with my google calendar |
access my to-do list from public computers |
Appendix B: Use Cases
For all use cases below, the System is 2Do
and the Actor is the user
, unless specified otherwise.
Use case: Display help guide
MSS
-
User requests for the help guide
-
System displays the help guide
Use case ends
Extensions
1a. User input is invalid
1a1. System displays an error message
Use case ends
Use case: Add a floating task
MSS
-
User requests to add a floating task with the name field filled and the end field empty
-
System adds the floating task and displays a success message
Use case ends
Extensions
1a. User input is invalid
1a1. System displays an error message
Use case ends
1b. User enters an alarm parameter using the natural language format
1b1. System displays an error message
Use case ends
Use case: Add a task with a deadline
MSS
-
User requests to add a task, with a deadline, with the name and end fields filled and the start field empty
-
System adds the task, with a deadline, and displays a success message
Use case ends
Extensions
1a. User input is invalid
1a1. System displays an error message
Use case ends
Use case: Add an event
MSS
-
User requests to add an event with the name, start and end fields filled
-
System adds the event and displays a success message
Use case ends
Extensions
1a. User input is invalid
1a1. System displays an error message
Use case ends
1b. User enters an end time that is earlier than the start time
1b1. System displays an error message
Use case ends
Use case: Add a tag
MSS
-
User requests to add a tag
-
System adds the tag and displays a success message
Use case ends
Extensions
1a. User input is invalid
1a1. System displays an error message
Use case ends
Use case: Delete task
MSS
-
User searches for a task using list or find
-
System displays all relevant tasks
-
User requests to delete the task by using its index
-
System deletes the task and displays a success message
Use case ends
Extensions
1a. User input is invalid
1a1. System displays an error message
Use case ends
3a. User input is invalid
3a1. System displays an error message
Use case resumes at step 2
Use case: Delete a tag
MSS
-
User requests to delete a tag
-
System deletes the tag and displays a success message
Use case ends
Extensions
1a. User input is invalid
1a1. System displays an error message
Use case ends
Use case: List tasks
MSS
-
User requests to list all tasks
-
System displays all tasks
Use case ends
Extensions
1a. User input is invalid
1a1. System displays an error message
Use case ends
Use case: Find task
MSS
-
User requests to find a task using a keyword
-
System displays all relevant tasks
Use case ends
Extensions
1a. User input is invalid
1a1. System displays an error message
Use case ends
Use case: Undo last action
MSS
-
User requests to undo the last action
-
System undoes the last action and displays a success message
Use case ends
Extensions
1a. User input is invalid
1a1. System displays an error message
Use case ends
2a. No actions were done previously
2a1. System displays an error message
Use case ends
Use case: Redo last undo command
MSS
-
User requests to redo the last undo command
-
System redoes the last undo command and displays a success message
Use case ends
Extensions
1a. User input is invalid
1a1. System displays an error message
Use case ends
2a. The last action was not an undo command
2a1. System displays an error message
Use case ends
Use case: Edit task
MSS
-
User searches for a task using list or find
-
System displays all relevant tasks
-
User requests to edit the task by using its index
-
System updates the task and displays a success message
Use case ends
Extensions
1a. User input is invalid
1a1. System displays an error message
Use case ends
3a. User input is invalid
3a1. System displays an error message
Use case resumes at step 2
Use case: Mark task as complete
MSS
-
User searches for a task using list or find
-
System displays all relevant tasks
-
User requests to mark the task as complete by index
-
System updates the task and displays a success message
Use case ends
Extensions
1a. User input is invalid
1a1. System displays an error message
Use case ends
3a. User input is invalid
3a1. System displays an error message
Use case resumes at step 2
3b. Task is already marked as complete
3b1. System displays an error message
Use case ends
Use case: Mark task as incomplete
MSS
-
User searches for a completed task using list /h or find /h
-
System displays all relevant tasks
-
User requests to mark the task as incomplete by index
-
System updates the task and displays a success message
Use case ends
Extensions
1a. User input is invalid
1a1. System displays an error message
Use case ends
3a. User input is invalid
3a1. System displays an error message
Use case resumes at step 2
3b. Task is already marked as incomplete
3b1. System displays an error message
Use case ends
Use case: Display options
MSS
-
User requests to view the general options available on system
-
System displays its general options
-
User requests to change the general options for the session
-
System updates its general options for the session and displays a success message
Use case ends
Extensions
1a. User input is invalid
1a1. System displays an error message
Use case ends
3a. User input is invalid
3a1. System displays an error message
Use case ends
3b. Options inputted are the same as the current general options
3b1. System displays an error message
Use case ends
Use case: Save to-do list
MSS
-
User requests to save the to-do list
-
System saves the current to-do list, exports it as an xml file in the directory specified and displays a success message
Use case ends
Extensions
1a. User input is invalid
1a1. System displays an error message
Use case ends
Use case: Load to-do list
MSS
-
User requests to load a to-do list xml file into the system
-
System loads the to-do list and displays the loaded to-do list
Use case ends
Extensions
1a. User input is invalid
1a1. System displays an error message
Use case ends
Use case: Clear to-do list
MSS
-
User requests to clear the to-do list
-
System clears the to-do list
Use case ends
Extensions
1a. User input is invalid
1a1. System displays an error message
Use case ends
Use case: Quit system
MSS
-
User requests to quit system
-
System shuts down
Use case ends
Extensions
1a. User input is invalid
1a1. System displays an error message
Use case ends
Appendix C: Non Functional Requirements
-
Should work on any mainstream OS as long as it has Java
1.8.0_60
or higher installed -
Should handle at least 100 tasks without a noticeable decline in performance
-
A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most tasks faster using commands than using the mouse
-
Should have automated unit tests and open source code
-
Should have user-friendly commands
-
Should not require more than 3 seconds to startup
-
Should not require more than 2 seconds to respond to a user request
-
Should not require internet connection
Appendix D: Glossary
Name
Name of task
Floating task
Task without an end time
Task with deadline
Task with an end time and without a start time
Event
Task with a start time and an end time
Recurring task
Task that repeatedly needs to be done every week, month or year
Index
Number assigned to a task to ease the operation of commands (E.g. add, delete, edit)
Last action
Last command executed
History
Completed tasks
Mainstream OS
Windows, Linux, Unix, OS-X
Appendix E: Product Survey
Google Calendar
Author: Ang Lang Yi
Pros:
-
User friendly commands, through simply filling its short and simple forms to handle tasks
-
Simple interface makes it easy to navigate around
-
Calendar interface allows the user to easily find empty slots to schedule tasks
-
Calendar can be viewed in a year, month, day or agenda format
-
Import and view other people’s calendars for better collaboration
-
Reminders can be set to a task
Cons:
-
Floating tasks are difficult to schedule
-
Navigation requires mouse usage instead of command line
-
Internet connection is required
Todoist
Author: Teo Shu Qi
Pros:
-
Features are intuitive as users simply need to fill in the short and simple forms provided to handle their tasks
-
Different categories are available to separate different kinds of tasks
-
Each category allows a daily and weekly view of its tasks
-
Recurring tasks can be added
-
Different priority levels can be set for tasks
-
Filter and find tasks according to priority level and floating tasks
Cons:
-
Only tasks due for the next 7 days can be seen
-
Some features (e.g. comments) require a monthly subscription
-
Navigation requires mouse usage instead of command line
-
Internet connection is required
Trello
Author: V Narendar Nag
Pros:
-
Multiple boards can be added to segregate different categories of tasks
-
Lists can be added to a board to separate that category into even more sections
-
A team can collaborate on a board together
-
Reminders can be set to a task
-
Comments can be added to a task for additional notes
-
Images and attachments can be added to a task
Cons:
-
Adding a task is not intuitive as its respective form is cluttered with additional features that makes it difficult to know which fields are mandatory
-
Setting up the calendar is dependent on another calendar application (e.g. Google Calendar) as it supports calendar import by other applications but lacks its own native calendar
-
Recurring tasks cannot be added
-
A command line interface is not available
-
Internet connection is required
Wunderlist
Author: Yogamurti Sutanto
Pros:
-
Short and simple forms are used to allow easy handling of tasks
-
Straightforward and uncluttered interface makes it easy for navigation
-
Various backgrounds are available to suit the user’s preferences
-
Sort uncompleted tasks in various ways
-
Tasks can be starred to indicate importance and segregate them from other tasks
-
Categories can be added to tasks for better organization
-
Multiple users can collaborate together on a particular category of the to-do list
-
Reminders can be set to a task
-
Subtask and notes can be added to a task
Cons:
-
A command line interface is not available
-
Internet connection is required